[Italian Version]
How I see/I like to see the application layers division? Every layer can speak only with the layer directly under of itself. Every layer has the knowledge of application models (business entities) and of course - for technical problems of circular reference -business entities don't know nobody.
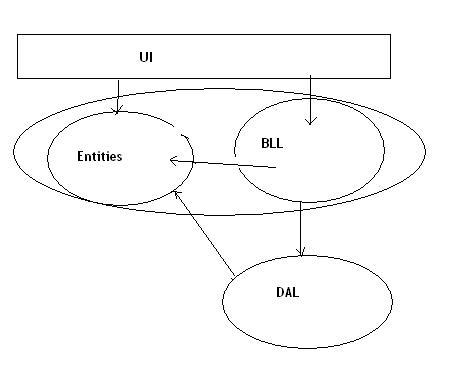
How implement a lazy load? Someone resolve the problem using a events system. The business entities notify the need to load data on demand. Interesting solution but I don't like that the entities know what needs to lazy load. The data load mode is - in my opinion - a DAL question. The DAL know the right way to load data. My conclusion is: polymorphism can be a good way!
It follow a raw code about my idea. I exclude the question of abstract DAL e concrete DAL... I have exposed already my opinion in the italian post "Archietture to plugIn, thoughts and considerations"...
[Assembly of Models (business entities)]
public class Person
{
private Guid code= Guid.Empty;
public virtual Guid Code
{
get{return code;}
set{code= value};
}
private string name = string.Empty;
public virtual string Name
{
get{return name;}
set{name = value};
}
private AddressCollection addresses = new AddressCollection ();
public virtual AddressCollection Addresses
{
get{return addresses ;}
set{addresses = value};
}
}
[Assembly of a concrete DAL]
class PersonDataProvider: IPersonDataProvider
{
public Person RetrievePersonByCode(Guid code)
{
//TODO: Prepare the command to retrieve person by code.
IDataReader r = cmd.OpenReader();
if(r.Read())
{
Person person = new InnerPerson();
person.Code = (Guid)r["Code"];
person.Name = (String)r["Name"];
person.Addresses = null;
return person;
}else{
return new UnknownPerson();
}
}
class InnerPerson: Person
{
public override AddressCollection Addresses
{
get
{
if(base.Addresses == null)
{
//TODO: prepare command to retrieve addresses of this person ...
//TODO: create and fill addresses collection ...
}
retturn base.Addresses;
}
set{base.Addresses = value;}
}
}
}
"You state that you want a function to have the flexibility of late-binding properties using the keyword virtual. You don’t need to understand the mechanics of virtual to use it, but without it you can’t do object-oriented programming in C++. In C++, you must remember to add the virtual keyword because, by default, member functions are not dynamically bound. Virtual functions allow you to express the differences in behavior of classes in the same family. Those differences are what cause polymorphic behavior." (Bruce Eckel, Thinking in C++)
posted @ venerdì 6 gennaio 2006 02:49