Immaginiamo di avere un database SQL Server esistente, e vogliamo utilizzare Entity Framework Code First per mappare il modello dati.
Supponendo di avere due tabelle “Items” e “Categories” in relazione come da Database Diagram seguente:
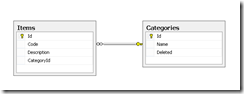
Ed una “Vista” SQL Server definita come segue:
CREATE VIEW [dbo].[VCategories]
AS
SELECT Id, Name
FROM Categories
WHERE (Deleted = 0)
Aggiungiamo due classi C# definite in questo modo:
public class Category
{
public int Id { get; set; }
public string Description { get; set; }
}
public class Item
{
public int Id { get; set; }
public string Code { get; set; }
public string Description { get; set; }
public int CategoryId { get; set; }
public Category Category { get; set; }
}
che mappiamo tramite OnModelCreating (nella classe derivata da DbContext) come segue :
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
modelBuilder.Entity<Category>().ToTable("VCategories")
.Property(c => c.Description).HasColumnName("Name");
modelBuilder.Entity<Item>().ToTable("Items");
modelBuilder.Entity<Item>().HasRequired(x => x.Category)
.WithMany().HasForeignKey(o => o.CategoryId);
}
Per verificare il funzionamento del mapping possiamo scrivere del codice tipo:
Item item = null;
using (Db db = new Db())
{
Console.WriteLine("Items count : {0}", db.Items.Count());
Category category = (from c in db.Categories where c.Id == 1 select c)
.FirstOrDefault();
item = new Item()
{
Category = category,
CategoryId = category.Id,
Code = "Code #1",
Description = "Description #1",
};
db.Items.Add(item);
db.SaveChanges();
Console.WriteLine("Items count : {0}", db.Items.Count());
}
using (Db db = new Db())
{
////Edit
item.Description = "New Description #1.";
db.Entry<Item>(item).State = System.Data.Entity.EntityState.Modified;
db.SaveChanges();
Console.WriteLine("Items count : {0}", db.Items.Count());
}
using (Db db = new Db())
{
////Delete.
db.Entry<Item>(item).State = System.Data.Entity.EntityState.Deleted;
db.SaveChanges();
Console.WriteLine("Items count : {0}", db.Items.Count());
}