Here’s a simple tutorial about using Expression Blend for HTML5 and some little Javascript to create a simple transition effect.
Fire up Expression Blend 5 and by double clicking the elements on Assets library add a button and a div, let’s rename it by double clicking them in LiveDOM pane.
Here’s live DOM contents and it’s HTML counterpart (I’ve manually added button Go! content)
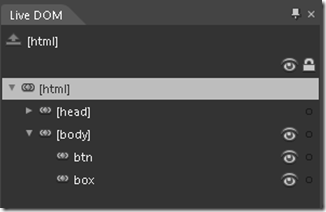 | <!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>WinJSTransitions</title> <!-- WinJS references --> <link rel="stylesheet" href="/winjs/css/ui-dark.css" /> <script src="/winjs/js/base.js"></script> <script src="/winjs/js/wwaapp.js"></script> <!-- WinJSTransitions references --> <link rel="stylesheet" href="/css/default.css" /> <script src="/js/default.js"></script> </head> <body> <button id="btn">Go!</button> <div id="box"></div> </body> </html>
|
It’s time to make the box div look like a rectangle by applying a style: Select box element inside Live DOM and click on the little down arrow inside CSS Properties tab and by using CSS Properties tab let’s add some style properties.
On right you see the Css that Blend generated while playing with properties (approach is very similar to Blend for XAML BTW…)
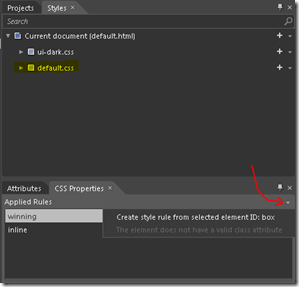 |
#box { border-radius: 20px; width: 200px; height: 200px; background-color: #4292B0; margin-top: 20px; }
|
Actual preview output
|
Since I want to use transition for margin-left and opacity properties let’s use Blend capability for this:
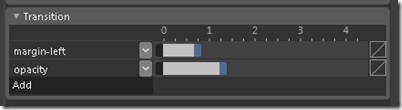 |
#box { border-radius: 20px; width: 200px; height: 200px; background-color: #4292B0; margin-top: 20px; -ms-transition-property: margin-left, opacity; -ms-transition-duration: 0.7s, 1.26s; -ms-transition-timing-function: linear, linear; }
|
As you see I’ve added the two properties inside Transition pane, set it’s duration by dragging the slider on right and set both to use linear easing, on right you can see how the css looks like now.
To trigger the transitions I’m going to dynamically add a class to box div, so let’s define them using Blend by first selecting the default.css file inside Styles tab then clicking on “+” button to create two class definition named “in” and “out” (see below)
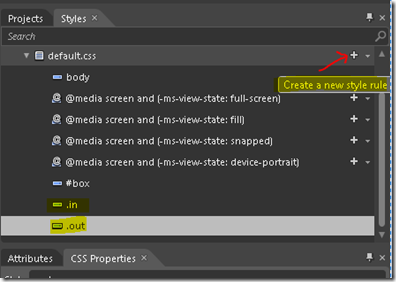
Now, with “in” class selected let change the value of opacity and margin-left to 1 and 0px respectively then select “out” class and change same properties to 0 and 300px, the related css entries should now look:
.in {
opacity: 1;
margin-left: 0px;
}
.out {
margin-left: 300px;
opacity: 0;
}
Let’s add the “in” class to our div by selecting it and typing “in” inside Attributes pane (manually editing the HTML is probably easier in this case…)
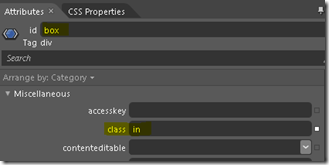
It’s now time to write to code (or should I say script?
) right click the project inside Blend and select “Edit in Visual Studio” to open the project in Visual Studio 2011
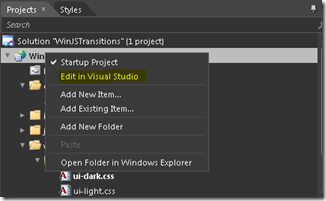
Code should look very straightforward: we’re going to subscribe to click event to add and remove “in” and “out” classes to the div element.
Before typing any code is necessary to add a reference to ui.js file from our HTML page because is there that addClass and removeClass methods live.
(function () {
'use strict';
// Uncomment the following line to enable first chance exceptions.
// Debug.enableFirstChanceException(true);
var isVisible=true;
WinJS.Application.onmainwindowactivated = function (e) {
if (e.detail.kind === Windows.ApplicationModel.Activation.ActivationKind.launch) {
document.getElementById("btn").addEventListener("click", function (e)
{
var box = document.getElementById("box");
var toAdd = isVisible ? "out" : "in";
var toRemove = isVisible ? "in" : "out";
WinJS.Utilities.removeClass(box, toRemove);
WinJS.Utilities.addClass(box, toAdd);
isVisible = !isVisible;
}, false);
}
}
WinJS.Application.start();
})();
Press F5 and you’ll see the rectangle moving and fading to the right and viceversa each time you click the button.
No rocket science here, but it works… 
Technorati Tags:
WinRT,
WinJS