Sometimes you might need to play multiple sounds at once (games are just one example) and as stated into documentation here: http://msdn.microsoft.com/en-us/library/ff426928(VS.96).aspx you can have only one MediaElement on a page, so: how do I play multiple sounds at the same time?
If you can afford to use plain 16 bit mono wav files you can use XNA’s SoundEffect class in just a few steps:
Add a project reference to Microsoft.Xna.Framework assembly.
Add the wav files to your project using Content as default build action (don’t forget that Resource is a load time performance hit)
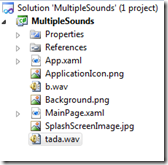
Then add following code inside App.xaml constructor:
readonly DispatcherTimer timer = new DispatcherTimer();
public App()
{
this.timer.Tick += (s, e) =>{FrameworkDispatcher.Update();};
this.timer.Interval = TimeSpan.FromMilliseconds(100);
this.timer.Start();
...
}
Finally load the sound you wish to play simultaneously using something like:
private void button1_Click(object sender, RoutedEventArgs e)
{
SoundEffect effect1 = SoundEffect.FromStream(TitleContainer.OpenStream("b.wav"));
SoundEffect effect2 = SoundEffect.FromStream(TitleContainer.OpenStream("tada.wav"));
effect1.Play();
effect2.Play();
}
and you’re done.
